Part I: Developing Inbound Applications - Application Menu
Suppose you want to give your customers the choice of whether
to use the voice commands version or the DTMF commands version.
With Voicent Gateway, you can easily add a web-based setup page to
your application. You can also add a custom menu to Voicent Gateway
for your application.
1. Create a setup jsp page
This page reads the user choice and saves the result to a file.
- <%@ page
import="java.io.FileWriter" %>
<%
response.setHeader("Cache-Control","no-cache");
String vcmd = request.getParameter("vcmd");
if (vcmd != null) {
String webappDir =
application.getRealPath("/");
FileWriter fw = new
FileWriter(webappDir + "/setup.txt");
fw.write(vcmd);
fw.close();
}
%>
<html>
<body>
<h1>Time of Day Service Setup</h1>
<a href="setup.jsp?vcmd=yes">Click here to use voice version</a>
<br>
<a href="setup.jsp?vcmd=no">Click here to use dtmf version</a>
</body>
</html>
Save the content to setup.jsp file under <tddir>/webapps.
2. Install the setup page
The steps are the same as installing the timeofday.jsp file.
- Compile the setup.jsp to setup.java using jspc
- Compile the setup.java to setup.class using
javac
- Add the following to web.xml
Add after other <servlet> definition
<servlet>
<servlet-name>setup</servlet-name>
<servlet-class>setup</servlet-class>
</servlet>
- Add after other <servlet-mapping> definition
- <servlet-mapping>
<servlet-name>setup</servlet-name>
<url-pattern>/setup.jsp</url-pattern>
</servlet-mapping>
Now, restart the gateway and test this page with a web browser.
The url should be
http://localhost:8155/td/setup.jsp
3. Use the setup information in timeofday.jsp
- <?xml
version="1.0"?>
<vxml version="1.0">
<%@ page import="java.util.Date" %>
<%@ page import="java.text.SimpleDateFormat" %>
<%@ page import="java.util.TimeZone" %>
<%@ page import="java.io.*" %>
- <%
response.setHeader("Cache-Control","no-cache");
Date d = new Date(System.currentTimeMillis());
String tz = request.getParameter("tz");
TimeZone timez = null;
if ("1".equals(tz) || "pacific time".equals(tz))
timez = TimeZone.getTimeZone("America/Los_Angeles");
else if ("2".equals(tz) || "mountain time".equals(tz))
timez = TimeZone.getTimeZone("America/Denver");
else if ("3".equals(tz) || "central time".equals(tz))
timez = TimeZone.getTimeZone("America/Chicago");
else if ("4".equals(tz) || "eastern time".equals(tz))
timez = TimeZone.getTimeZone("America/New_York");
-
SimpleDateFormat f;
-
if (timez != null) {
f = new SimpleDateFormat("hh:mm a,
zzzz");
f.setTimeZone(timez);
}
else {
f = new SimpleDateFormat("hh:mm a");
}
-
boolean useVcmd = false;
try {
String webappDir =
application.getRealPath("/");
FileReader fr = new
FileReader(webappDir + "/setup.txt");
BufferedReader br = new
BufferedReader(fr);
String line = br.readLine();
if ("yes".equals(line))
useVcmd =
true;
}
catch (IOException e) {
}
%>
<form
id="td">
<field name="tz">
<prompt timeout="10s">
<block>
<audio src="audio/welcome.wav"/>
<%= f.format(d) %>
<% if (useVcmd) { %>
<audio src="audio/which_timezone.wav"/>
<% } else { %>
<audio src="audio/which_timezone_dtmf.wav"/>
<% } %>
</block>
</prompt>
-
<% if (useVcmd) { %>
<grammar>
pacific time | mountain time | central time |
eastern time
</grammar>
<% } else { %>
<dtmf>
1 | 2 | 3 | 4
</dtmf>
<% } %>
<filled>
<submit next="/td/timeofday.jsp" namelist="tz"/>
</filled>
</field>
</form>
- </vxml>
Compile and jsp file and generated java file.
3. Install Application Menu to Voicent Gateway
Add the following line to timeofday.conf file.
- ctxmenu =
TimeOfDay ...
ctxmenulocalurl = td/setup.jsp
Reinstall the timeofday.conf file. Now you should see the new
menu is added to Voicent Gateway's Application main menu. You can
also use right mouse click on the gateway icon to access the
application menu.
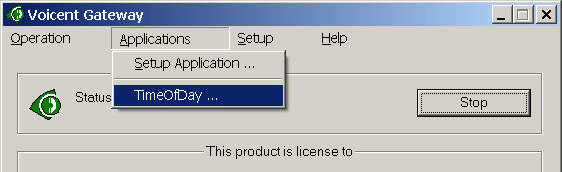
Download the sample code: timeofday_step6.zip.